TM1637 based display:
Driver:
gitlab.com
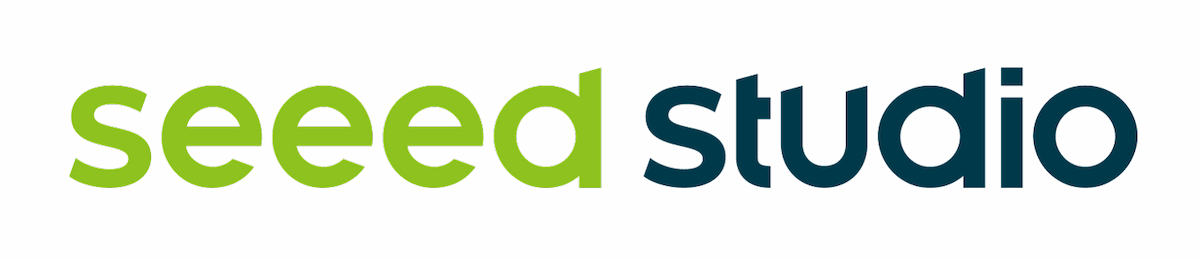
Driver:
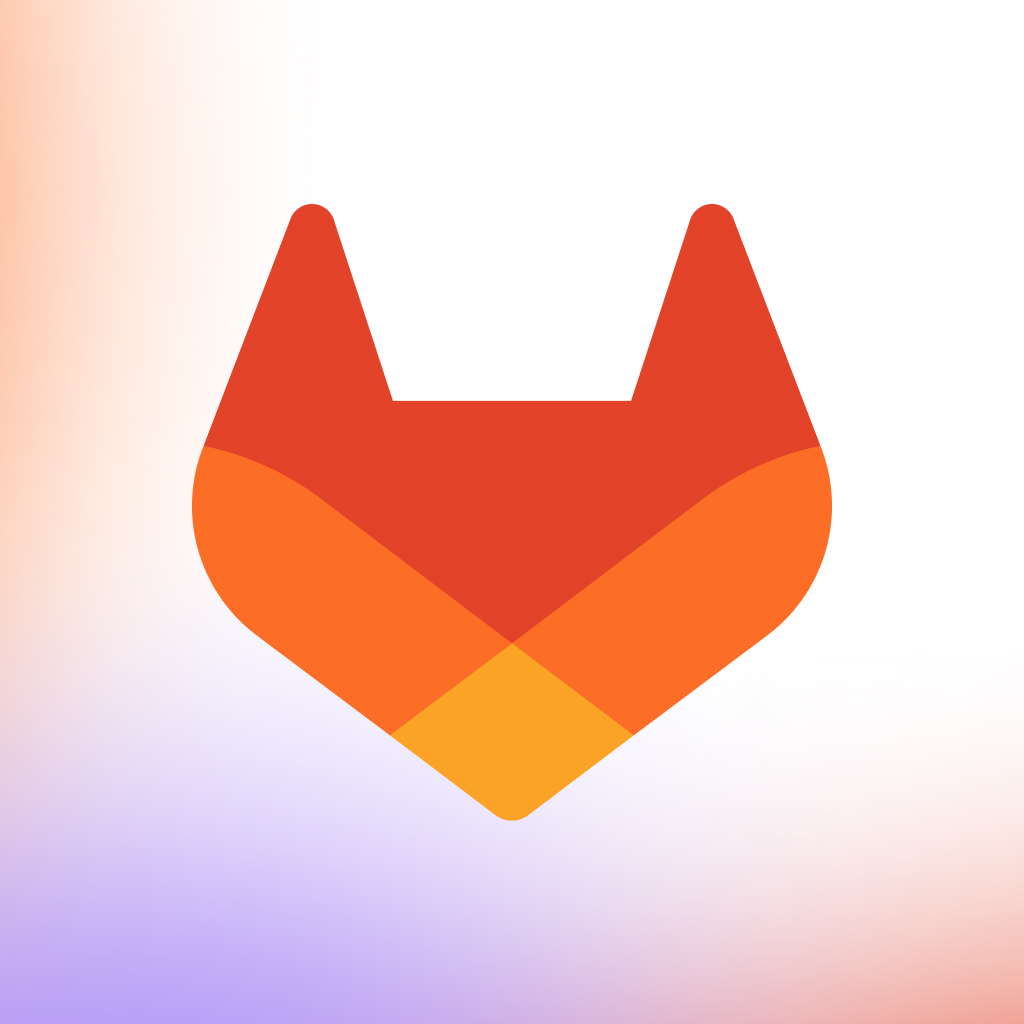
Александр Мишин / tm1637-display · GitLab
A FreeBSD version of a tm1637-display library written by Fred.Chu for Arduino. Added a lot of functions for display custom symbols and int values by AlexGyver. Adapted for...
